R3 Draw - Shape Sub-Commands
Contents | ||
See also:
- R3 View - Graphics Draw Dialect
- R3 Draw - Setup and Attribute Commands
- R3 Draw - Line Related Commands
- R3 Draw - Curve Related Commands
Concept
This section describes special commands optimized for describing shapes. All of these commands are used withing the shape argument block and should not be used separately.
Arc
Draws an elliptical arc from the current point.
Argument | Datatype | Description |
---|---|---|
end-point | pair! | |
radius-x | decimal! | |
radius-y | decimal! | |
angle | decimal! | |
sweep | word! | the arc will be drawn in a "positive angle" direction sweep |
large | word! | one of the large arc sweeps will be chosen large |
Simple arc example:
pen yellow line-width 3 shape [ move 100x200 arc 300x200 120 50 0 ]
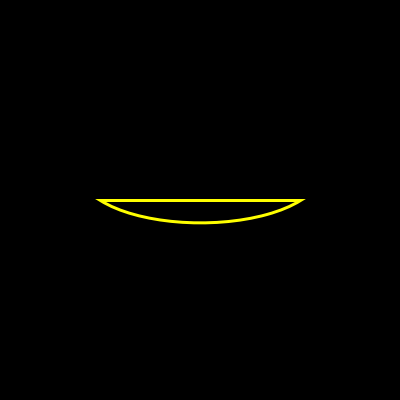
arc example 1
Set SWEEP to draw arcs in a "positive-angle" direction:
pen yellow line-width 3 shape [ move 100x200 arc 300x200 120 50 0 sweep ]
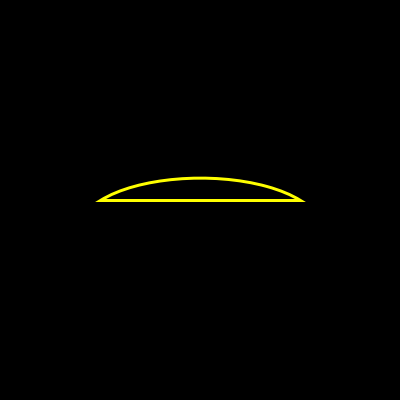
arc example 2
Set LARGE for arc sweeps greater than 180 degrees:
pen yellow line-width 3 shape [ move 100x200 arc 300x200 120 50 0 sweep large ]
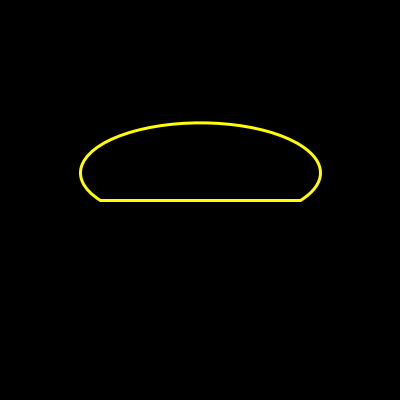
arc example 3
More complex usage of arc:
pen yellow line-width 3 shape [ move 0x399 line 42x357 arc 84x315 25 20 -45 sweep line 126x273 arc 168x231 25 40 -45 sweep line 210x189 arc 252x147 25 60 -45 sweep line 294x105 arc 336x63 25 80 -45 sweep line 399x0 move 0x0 ]
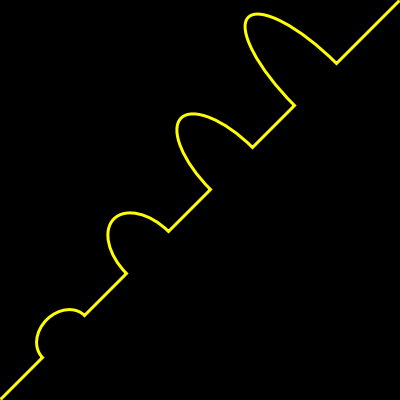
arc example 4
Same complex example but with relative positioning commands:
pen red line-width 3 translate 0x399 shape [ move 0x0 'line 42x-42 'arc 42x-42 25 20 -45 sweep 'line 42x-42 'arc 42x-42 25 40 -45 sweep 'line 42x-42 'arc 42x-42 25 60 -45 sweep 'line 42x-42 'arc 42x-42 25 80 -45 sweep 'line 63x-63 move 0x0 ]
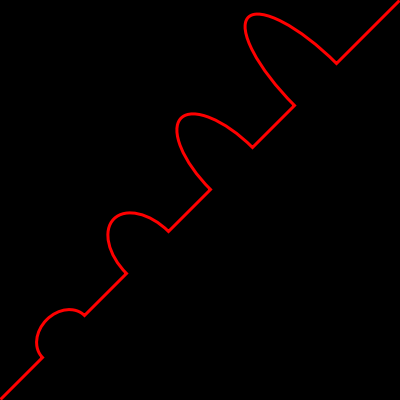
arc example 5
Close
Closes previously defined set of lines in the SHAPE block.
This command has no arguments.
In some case it is easier to use the CLOSE command as a shortcut instead of calculating the closing lines.
This is without CLOSE commands:
line-width 3 pen yellow shape [ move 100x100 hline 50 arc 100x50 50 50 0 large move 75x75 vline 25 arc 25x75 50 50 0 move 0x160 line 200x160 200x0 0x0 ]
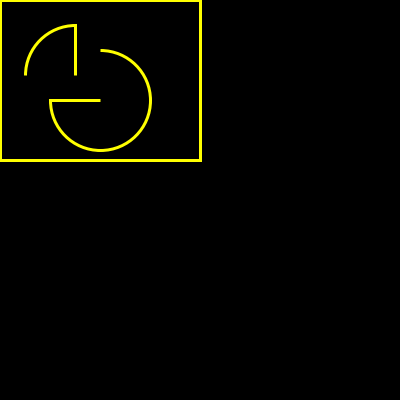
close example 1
And here with CLOSE commands:
line-width 3 pen yellow shape [ move 100x100 hline 50 arc 100x50 50 50 0 large close move 75x75 vline 25 arc 25x75 50 50 0 close move 0x160 line 200x160 200x0 0x0 ]
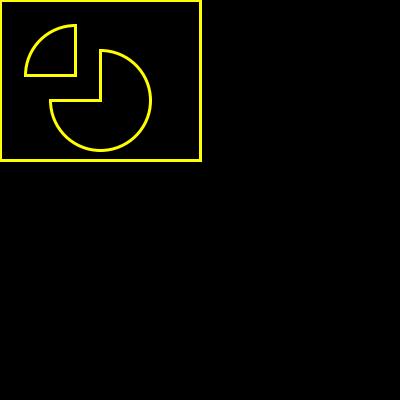
close example 2
Curv
Smooth CURVE command shortcut.
Argument | Datatype | Description |
---|---|---|
point1 | pair! | |
point2 | pair! | |
point1 | pair! | |
... | pair! |
From http://www.w3.org/TR/SVG11/paths.html:
"The first control point is assumed to be the reflection of the second control point on the previous command relative to the current point. (If there is no previous curve command, the first control point is the current point.)"
Simple example:
line-width 3 pen yellow shape [ move 100x50 vline 150 curv 300x150 300x50 move 0x0 ]
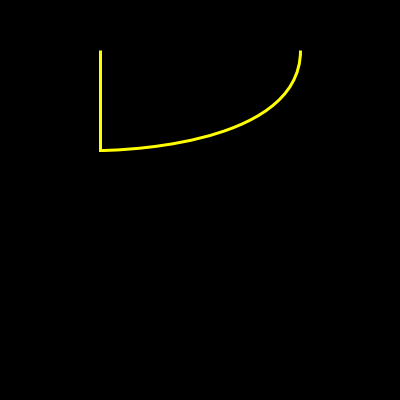
curv example 1
Same example but with relative positioning commands:
line-width 3 pen red shape [ 'move 100x50 'vline 100 'curv 200x0 200x-100 move 0x0 ]
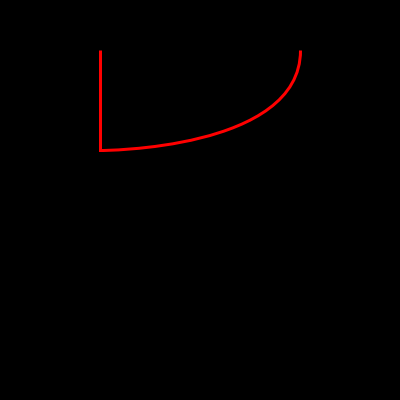
curv example 2
Curve
Draws a cubic Bézier curve.
Argument | Datatype | Description |
---|---|---|
point1 | pair! | |
point2 | pair! | |
point3 | pair! | |
point4 | pair! | |
... | pair! |
A cubic Bézier curve is defined by a start point, an end point, and two control points.
line-width 3 pen yellow shape [ move 100x50 curve 100x150 300x150 300x50 move 0x0 ]
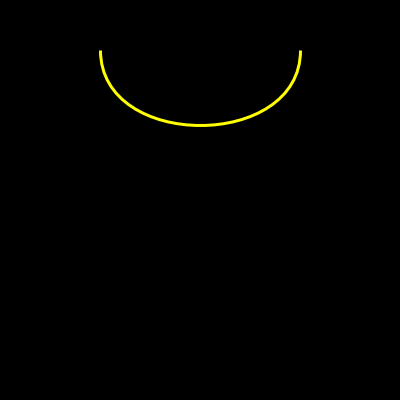
curve example 1
Same example but with relative positioning commands:
line-width 3 pen red shape [ move 100x50 'curve 0x100 200x100 200x0 move 0x0 ]
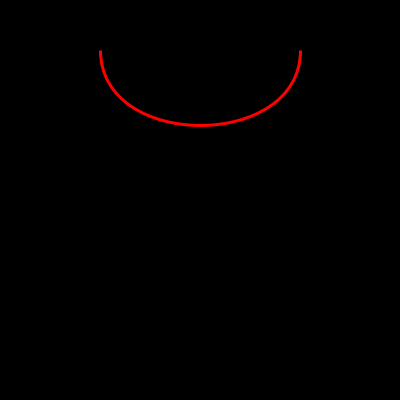
curve example 2
Hline
Draws a horizontal line from the current point.
Argument | Datatype | Description |
---|---|---|
end-x | decimal! |
Using absolute coordinates:
pen yellow line-width 4 shape [ move 100x100 hline 300 move 100x150 hline 250 move 100x200 hline 200 ]
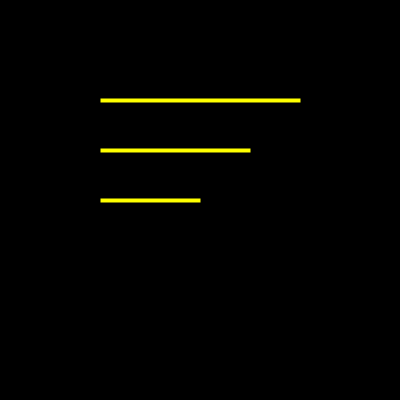
hline example 1
Using relative coordinates:
pen red line-width 4 shape [ move 100x100 'hline 200 'move -200x50 'hline 150 'move -150x50 'hline 100 ]
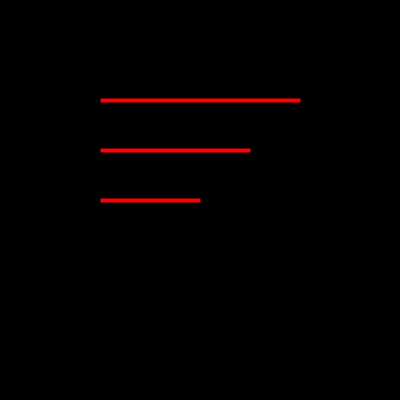
hline example 2
Line
Draws a line from the current point through the given points, the last of which becomes the new current point.
Argument | Datatype | Description |
---|---|---|
point1 | pair! | |
point2 | pair! | |
point3 | pair! | |
point4 | pair! | |
... | pair! |
Using absolute coordinates:
pen yellow line-width 4 shape [ move 50x50 line 300x120 50x120 300x50 move 0x0 ]
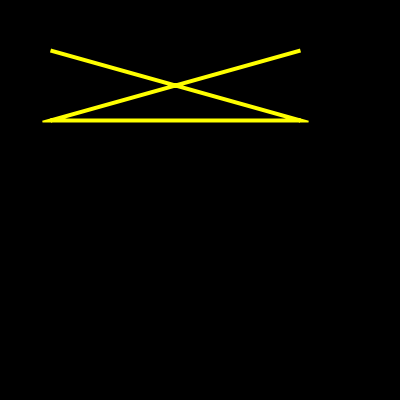
line example 1
Using relative coordinates:
pen red line-width 4 shape [ move 50x50 move 50x50 'line 250x70 -250x0 250x-70 move 0x0 ]
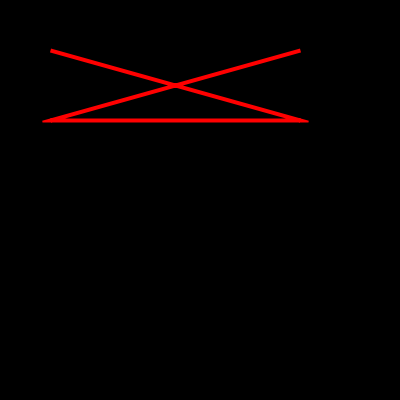
line example 2
Move
Set's the starting point for a new path without drawing anything.
Argument | Datatype | Description |
---|---|---|
point1 | pair! |
The effect is as if the "pen" were lifted and moved to a new location.
Used at the end of a SHAPE command, MOVE prevents the shape from being drawn as a closed polygon.
Note: Every path defined in SHAPE block is automatically closed. To disable the auto-close feature just put at the end of the SHAPE block: move 0x0
line-width 4 pen red shape [ move 100x100 line 20x20 150x50 move 0x0 ] pen blue shape [ move 100x200 line 20x120 150x150 ]
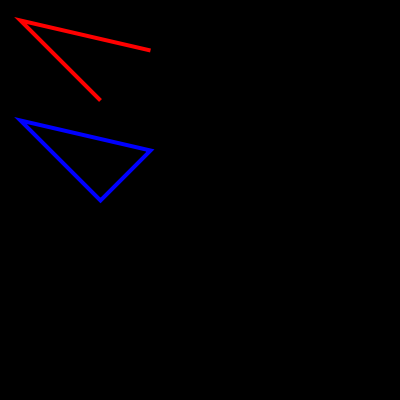
move example 1
Using relative coordinates for the second shape:
line-width 4 pen red shape [ move 100x100 line 20x20 150x50 move 0x0 ] pen blue shape [ move 100x100 'move 0x100 'line -80x-80 130x30 'move 0x0 ]
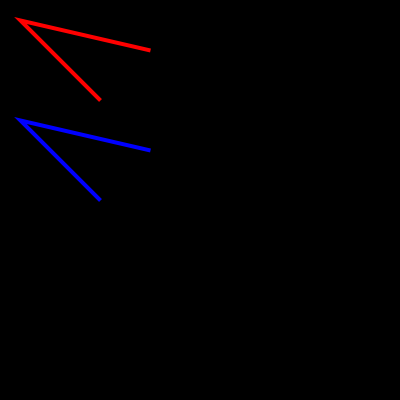
move example 2
Qcurv
Smooth QCURVE command shortcut.
Argument | Datatype | Description |
---|---|---|
point1 | pair! |
Draws a cubic Bézier curve from the current point to point1.
See: http://www.w3.org/TR/SVG11/paths.html and CURV
Using absolute coordinates:
pen yellow line-width 4 shape [ move 0x150 qcurve 100x250 200x150 qcurv 400x150 move 0x0 ]
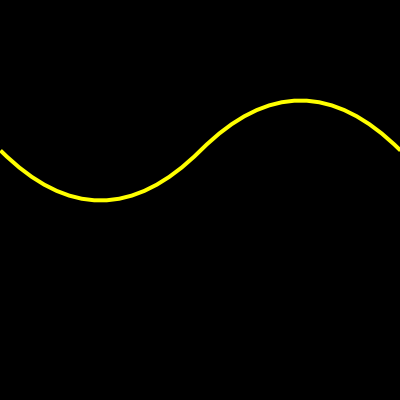
qcurv example 1
Using relative coordinates:
pen red line-width 4 shape [ move 0x150 'qcurve 100x100 200x0 'qcurv 200x0 move 0x0 ]
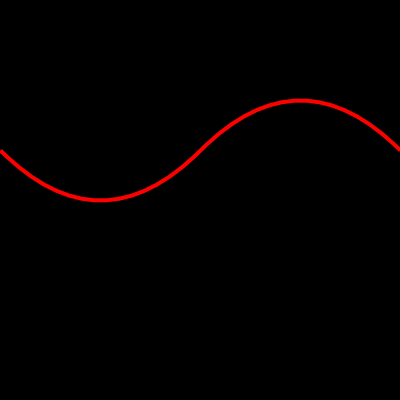
qcurv example 2
Qcurve
Draws quadratic Bézier curve.
Argument | Datatype | Description |
---|---|---|
point1 | pair! | |
point2 | pair! | |
point3 | pair! | |
... | pair! |
A quadratic Bézier curve is defined by a start point, an end point, and one control point.
Using absolute coordinates:
pen yellow line-width 4 shape [ move 100x50 qcurve 200x150 300x50 move 0x0 ]
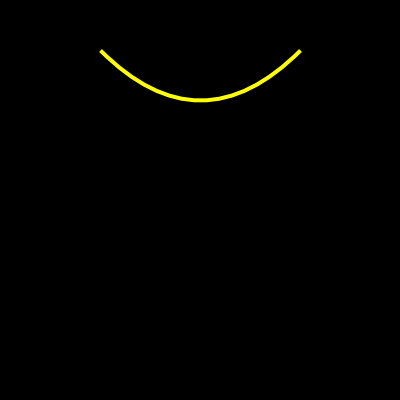
qcurve example 1
Using relative coordinates:
pen red line-width 4 shape [ move 100x50 'qcurve 100x100 200x0 move 0x0 ]
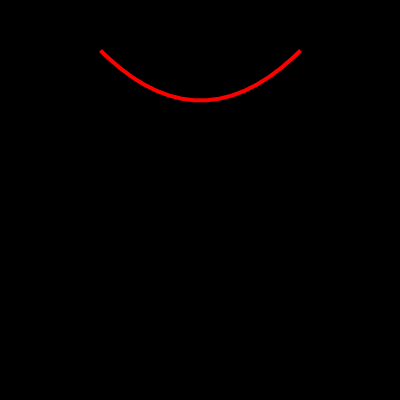
qcurve example 2
Vline
Draws a vertical line from the current point.
Argument | Datatype | Description |
---|---|---|
end-y | decimal! |
Using absolute coordinates:
pen yellow line-width 4 shape [ move 100x100 vline 300 move 150x100 vline 250 move 200x100 vline 200 ]
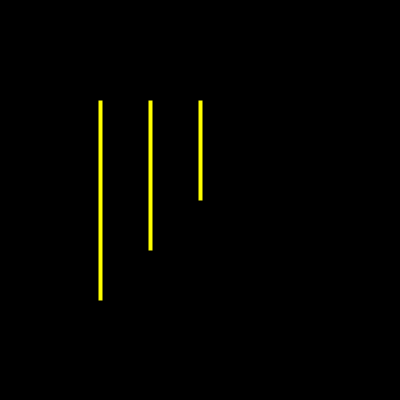
vline example 1
Using relative coordinates:
pen red line-width 4 shape [ move 100x100 'vline 200 'move 50x-200 'vline 150 'move 50x-150 'vline 100 ]
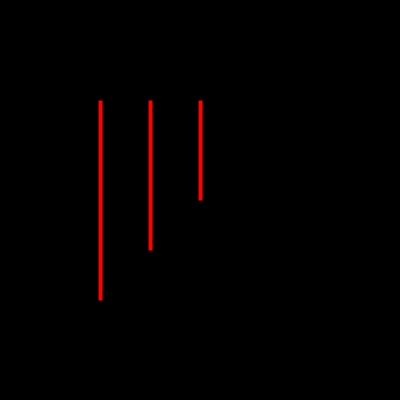
vline example 2