R3 GUI - User's Guide
Contents | ||
REBOL's Designer
Revised: 16-Mar-2010
Return to: R3 Graphical User Interface (GUI)
Introduction
This page introduces the main concepts of the REBOL 3 graphical user interface, the GUI.
In the sections below, I will show you how to quickly get started making your own windows with text, buttons, fields, panels, and many other types of GUI object. Although this document is intended for beginners, experienced REBOL programmers may find it a useful introduction as well.
Although the general principles of this GUI are similar to those of REBOL 2, it's been through a major redesign to incorporate all of what we've learned over the years in writing code the REBOL way.
Specifically, we designed a GUI that is:
Powerful | Can create a wide range of GUIs for our REBOL applications. |
Clean | Easier to read with less clutter that other languages or methods. |
Simpler | Much less complex both for apps and for the system itself. |
Fast | Quick to build and/or modify user interfaces. |
Pretty | Updated display method with anti-aliased scalar vector graphics (SVG). |
Hello World in a Window
These lines will open a new window, display some text, and provide a close button:
view [ title "Hello World" button "Close" close ]
When you run it, it looks like this:
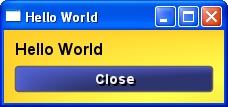
Hello world window (alpha skin)
Clicking the button will close the window.
Note that the bright background color indicates this is the alpha test version of the GUI. This will not be the final release default colors.
Don't Forget...
In order to run the above example, be sure to add these lines to the top:
REBOL [] load-gui
The load-gui function will download the current under-development GUI system. Later, this line will not be needed.
What's Going On?
In this example, the view function opens window and displays a panel. The panel was created from a layout block that includes two styles and their options:
Style | Description |
---|---|
title | A style that displays a title in a large bold font at the top of the panel. It also sets the window title to the same string. |
button | A style that when clicked will do some kind of action. Here it close the window, and because no other GUI windows are open, REBOL will quit too. |
In this example, the options for those styles are the strings that follow them, and for the button, the the word close is a special kind of reactor option.
Notice that we call graphic elements styles, not controls, widgets, or gadgets. That's because they aren't hard coded functions as found in most GUI systems; they are more like the style sheets, a bit like CSS used for web pages.
Simple Forms
To show how quickly you can build user interfaces, I'll start by making a few input forms.
Basic Form
Let's expand on the example above by making it into a small input form.
This window:
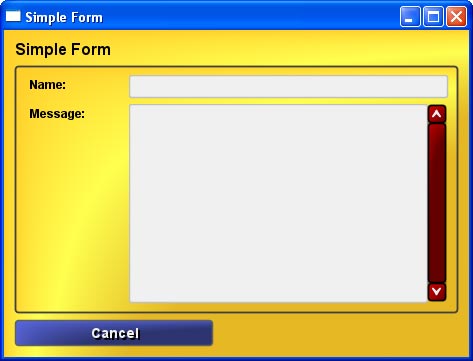
Simple form window (alpha skin)
is created from this code:
view [ title "Simple Form" panel 2 [ label "Name:" field label "Message:" area ] button "Cancel" close ]
And, I've introduced a few new styles:
Style | Description |
---|---|
panel | Creates sub-panel with two columns (from the integer that follows it). |
label | Text labels for name and message. |
field | A single-line text input field. |
area | A multiple-line text input field. |
A Real Form
The above form, although it looks good, doesn't do anything useful. So, let's add a couple more buttons to make the form do something.
I will add two more buttons: one for submit and the other for help:
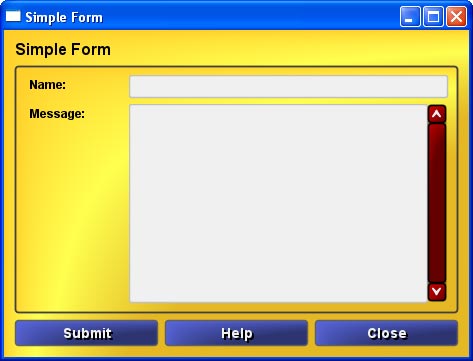
Form with submit and help (alpha skin)
This window comes from the code:
view [ title "Simple Form" panel 2 [ label "Name:" name: field label "Message:" message: area ] group [ button "Submit" submit http://www.rebol.com/cgi-bin/gui-form.r button "Help" browse http://www.rebol.com/r3/docs/gui/gui.html button "Close" close ] ]
Notice the group style. It's similar to a panel but has no borders and places items horizontally. It turn out that these two styles are related. One is defined from the other, but I'll talk more about that later.
The submit button will automatically submit all the input fields to the given URL. I will be talking about this more later. On submit, the data of the fields are formatted like an object, with names and values. That's why I've put set-word names in front of the field and area styles.
The help button will open the default web browser to the given URL. Easy.
Survey Form
Next, I'm adding more to the above form to make an opinion survey out of it:
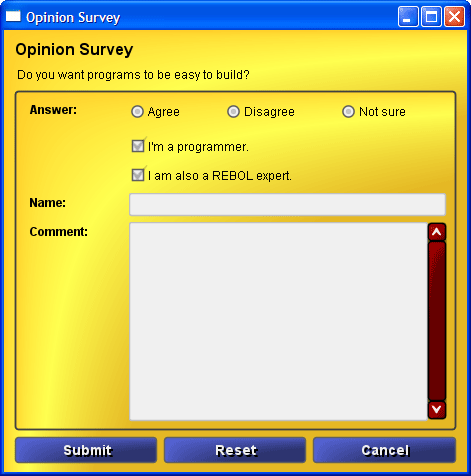
Opinion survey form (alpha skin)
You can see I've added a few more styles here:
view [ title "Opinion Survey" text "Do you want programs to be easy to build?" panel 2 [ label "Answer:" group [ radio "Agree" radio "Disagree" radio "Not sure" ] pad check "I'm a programmer." pad check "I am also a REBOL expert." label "Name:" field label "Comment:" area pad ; temporary, for bug ] group [ button "Submit" submit http://www.rebol.net/cgi/submit.r button "Reset" reset button "Cancel" close ] ]
The new styles in this example are:
Style | Description |
---|---|
text | Displays simple text lines |
radio | Mutually exclusive choice buttons (classic radio buttons). |
pad | A filler for an empty position in the panel. |
check | A check box button and text. |
In addition, the second button performs a reset action on the form, resetting all contents to its initial values.
Also, you may be wondering why there are no set-word names for the various input fields. Yes, that's accepted, and I'll talk more about that when I cover how submit works.
What's Missing
Something's missing in the above forms. Did you notice?
They end with the user submitting data to a URL, but then what? Shouldn't we see a response from the server, some kind of confirmation or perhaps even the current results of the survey? Yes, indeed we need that, and I'll be showing that in the sections ahead.
Other Input Styles
Here's a fun one to try. This window will show a few other input styles and the top two bars will change based on changes you make to the other bars or buttons that you click.
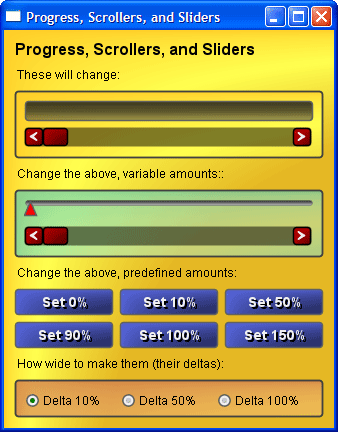
Other input styles (alpha skin)
The check boxes as the bottom will determine the width of the scroll bar knob.
It all comes from this code:
view [ title "Progress, Scrollers, and Sliders" text "These will change:" panel [ prog: progress sbar: scroller attach 'prog ] text "Change the above, variable amounts::" panel 80.200.180.80 [ slider attach 'sbar scroller attach 'sbar ] text "Change the above, predefined amounts:" group 3 [ button "Set 0%" set 'sbar 0% button "Set 10%" set 'sbar 10% button "Set 50%" set 'sbar 50% button "Set 90%" set 'sbar 90% button "Set 100%" set 'sbar 100% button "Set 150%" set 'sbar 150% ] text "How wide to make them (their deltas):" panel 3 200.100.80.80 [ radio "Delta 10%" on set 'sbar 'delta 10% radio "Delta 50%" set 'sbar 'delta 50% radio "Delta 100%" set 'sbar 'delta 100% ] ]
These new styles are being used:
Style | Description |
---|---|
progress | Displays a progress bar. |
scroller | A sliding scroll bar with arrow buttons. |
slider | A sliding control bar. |
In addition, these two reactors make it all work:
Reactor | Description |
---|---|
attach | Attach one GUI element to another. It's value will automatically set the other value. |
set | Set the value of a GUI element and update its display. |
This is a good time to mention that in R3 a GUI element is called a face (as in interface or surface.) A face is an instance of a style. It's the actual object created in the panel that holds the attributes and values related to the style.
More to come...
This is just a start. I'll be adding more very soon.
-Carl March 2010