REBOL/View Graphics - Face Effects
Contents | ||
Dynamic modifications that can be made to faces.
Face Effects
Within a face, the effect field can be set to a word! or a block! that describes image processing operations to perform on the backdrop of a face. When a block! is used, multiple effects can be specified; they are applied in the order in which they appear within the block. A wide range of effects can be produced with effects (there are over 30 individual effects that can be combined in any number and sequence).
Merging Background
It was necessary to add the merge effect on newer versions of REBOL in order to explicitly determine when an effect involves its background.
Effect | Arguments | Description |
---|---|---|
merge | none | Merges the bitmaps of faces that fall behind the current face into the current effect. This is how an effect can be applied to areas of the background. |
The code below shows the merge effect in action. The first face object (the "kid" image) is shown both with and without merge. Below it are two other effect faces that are merged. Try deleting the merge words to see what happens.
palms: load-thru/binary http://data.rebol.com/view/palms.jpg kid: load-thru/binary http://data.rebol.com/docs/graphics/kid.png view f: make face [ offset: 100x100 size: palms/size image: palms text: "" effect: [gradcol 0x1 0.0.255 255.0.0] pane: reduce [ make face [ offset: 90x20 size: kid/size edge: none image: kid ] make face [ offset: 160x20 size: kid/size edge: none image: kid effect: [merge] ] make face [ offset: 0x110 size: palms/size * 1x0 + 0x10 edge: none effect: [merge gradmul black white] ] make face [ offset: 0x130 size: palms/size * 1x0 + 0x40 edge: none effect: [merge invert] ] ] ]
The resulting image is:
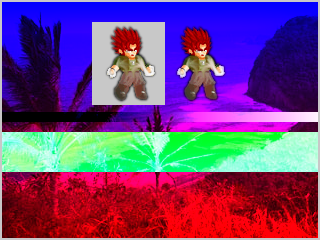
Older Scripts - If you run some older REBOL scripts and the effect is not appearing correctly, usually it is just a matter of adding the word merge to the head of the effect block.
Scaling
These effects control how the bitmap within the face is scaled relative to the face's size field.
Effect | Arguments | Description |
---|---|---|
fit | none | Scales an image to the size of the face, less the edge of the face if it exists. The image will be scaled both horizontally and vertically to fit within the face. |
aspect | none | Similar to fit, but preserves the perspective of the image. The image is not distorted. If the image does not span the entire face, the remaining portion will be filled with the face background color. |
extend | pair! pair! | Extends an image horizontally, vertically, or both. An image is stretched without affecting its scale. For instance, a button with rounded ends can be resized without affecting the dimensions of the rounded ends. This allows a single button bitmap to be reused over a wide variety of sizes. Two pair values are supplied as arguments. The first pair specifies the offset where the image should be extended. It can be horizontal, vertical, or both. The second pair specifies the number of pixels to extend in either or both directions. |
Here is an example that shows how the scaling effects can be used on an image:
butn: load-thru/binary http://data.rebol.com/how-to/graphics/button.gif btn-face: make face [ edge: none color: none image: butn ] view f: make face [ offset: 100x100 size: 400x60 color: white pane: reduce [ make btn-face [ offset: 10x10 size: butn/size ] make btn-face [ offset: 80x10 size: 130x38 effect: [fit] ] make btn-face [ offset: 210x20 size: 40x40 effect: [aspect] ] make btn-face [ offset: 255x10 size: 120x40 effect: [extend 35x0 50x0] ] ] ]
The result is:

Tiling
Images can be tiled within a face. The locations of the tiles can be face-relative or window-relative.
Effect | Arguments | Description |
---|---|---|
tile | none | Repeats the image over the entire face. This allows you to apply textures that span an entire face. The tile offset will be relative to the face. |
tile-view | none | Similar to tile, but the tile offset will be relative to the window face. |
Subimages
These effects are provided for times when only part of an image is needed.
Effect | Arguments | Description |
---|---|---|
clip | none | Clips an image to the size of the face. This is normally done when the image is larger than the face, and the remaining effects do not need to be performed on the entire bitmap. The clip can be done at anytime in the effect block. For instance a clip done before a flip will produce a different result than a clip done after a flip. |
crop | pair! pair! | Extracts a portion of an image. This effect takes two pair! values: the offset into the image and the size of the area needed. This operation can be used to pick any part of an image to be displayed separately. It allows you to pan and zoom on images. |
Rotation, Reflection, Flipping
These effects control various types of rotation, reflection, and flipping.
Effect | Arguments | Description |
---|---|---|
flip | pair! | Flips an image vertically, horizontally, or both. A pair! is provided as an argument to specify the direction of the flip. The X specifies horizontal and the Y specifies vertical. |
rotate | integer! | Rotates an image. An integer! specifies the number of degrees to rotate in the clockwise direction. (Currently only 0, 90, 180, and 270 degree rotations are supported.) |
reflect | pair! | Reflects an image vertically, horizontally, or both. A pair! is used to indicate the direction of reflection. The X value will reflect horizontally, and the Y value will reflect vertically. Negative and positive values specify which portion of the image is reflected. |
Image Processing
These are mostly color-oriented processing effects.
Effect | Arguments | Description |
---|---|---|
invert | none | Inverts the RGB values of an image. (Inversion is in the RGB color space.) |
luma | integer! | Lightens or darkens an image. An integer! specifies the degree of the effect. Positive values lighten the image and negative values darken the image. |
contrast | integer! | Modifies the contrast of the image. An integer! specifies the degree of the effect. A positive value increases the contrast and a negative value reduces the contrast. |
tint | integer! | Changes the tint of the image. An integer! specifies the color phase of the tint. |
grayscale | none | Converts a color image to black and white. |
colorize | tuple! | Colors an image. A tuple! specifies the color. The image is automatically converted to grayscale before it is colorized. [???Note] in docs/view-face-effects.txt |
multiply | tuple! | Multiplies each RGB pixel of an image to produce interesting coloration. An integer!, tuple!, or image! can be specified. An integer! will multiply each color component of each pixel by that amount (a value of 128 equals a multiplier of 1). A tuple! will multiply each of the red, green, and blue components separately. An image! will multiply the red, green, and blue components of an image, allowing you to apply textures to existing images. To divide, use values less than 128 for a color component. For example, do divide a color by two, use a value of 64. |
difference | tuple! | Computes a difference of RGB pixel values. This can be used to compare two images to detect differences between them. An image! is provided as an argument. Each of its RGB pixel values will be subtracted from the face image. |
blur | none | Blurs an image. This effect may be used multiple times to increase the effect. |
sharpen | none | Sharpens an image. This effect may be used multiple times to increase the effect. |
emboss | none | Applies an emboss effect to the image. The image is converted to grayscale and edges are highlighted. This effect may be used multiple times; the definition decreases with each application. |
Gradients
A gradient of colors can be added to faces with these effects. Note that the draw effect provides for a greater range of gradient patterns. (Eventually to be added here too.)
Effect | Arguments | Description |
---|---|---|
gradient | pair! tuple! tuple! | Generates a color gradient. A pair! and two color tuple! values can be supplied as arguments (optional). The pair! is used to determine the direction of the gradient. The X value of one specifies horizontal and a Y value of one specifies vertical. Both X and Y can be specified at the same time, producing a gradient in both directions. Negative values reverse the gradient in that direction. |
gradcol | pair! tuple! tuple! | Colorizes an image to a gradient. Arguments are identical to gradient. The image is colorized according to the colors of the gradient. |
gradmul | pair! tuple! tuple! | Multiplies an image over a gradient. Arguments are identical to gradient. The image is multiplied according to the colors of the gradient. |
Keys
Special types of color keys to support transparent effects when the image does not provide an alpha channel.
Effect | Arguments | Description |
---|---|---|
key | tuple! | Creates a transparent image by keying. A tuple! or integer! can specify a chroma or luma key effect. A tuple! will cause all pixels of the same value to become transparent. An integer! will cause all pixels with lesser luma values to become transparent. |
shadow | pair! | Creates a drop shadow on a keyed image. Accepts the same arguments as key, but in addition to a creating transparent image it generates a 50 percent drop shadow. |
Algorithmic Shapes
A few shapes can be algorithmically generated to reduce the need for images in some frequently used cases.
Effect | Arguments | Description |
---|---|---|
draw | block! | Renders scalar vector graphic (SVG) descriptions into images. This allows drawing of lines, shapes, fills and much more. See the Draw Dialect document for complete information. |
arrow | tuple! decimal! | Generate an arrow image. (Note: to get a better arrow, use the draw effect). An optional tuple! can be used to specify the color of the arrow, otherwise the edge color will be used. The arrow is proportional to the size of the face times an optional decimal value that can be provided. The direction of the arrow can be altered with flip or rotate. |
cross | tuple! | Generate an X cross image. (Note: see draw effect to get a better X.) This is used for check boxes. An optional tuple! can be used to specify the color of the cross, otherwise the edge color will be used. The cross is proportional to the size of the face. |
oval | tuple! | Generate a oval image. (Note: see draw effect to get a better oval.) An optional tuple! can be used to specify the color of outside of the oval, otherwise the edge color will be used. The oval will be proportional to the size of the face. |
round | pair! tuple! integer! integer! | Generate tab buttons with rounded corners. The optional arguments are: a pair! that specifies the edge to round, a tuple! that is used as an edge color, an integer! that indicates the radius of the curves, and an integer! that controls the thickness of the edge. |
grid | pair! pair! tuple! integer! pair! | Generate a two dimensional grid of lines. This is a useful backdrop for graphical layout programs. The optional arguments are: a pair! that specifies the horizontal and vertical spacing of the grid lines, a pair! that specifies the offset of the first lines, a pair! that indicates the thickness of the horizontal and vertical lines, and a tuple! that provides the color of the lines. |
Pending Docs
PENDING! Need docs for these. Some of these may not even be supported: add, mix, shadow, chisel, hsv, anti_alias, alphamul